Area of Interest & Multi-Peer

Overview
This sample project demonstrates the use of two core features of Photon Fusion: Area of Interest & Multi-Peer Mode.
Area of Interest is one of several types of interest management that Fusion offers. Area of Interest (AoI) can help with optimization in applications that have a lot of NetworkObjects that the player does not need to process all the same time. This is accomplished by assigning a radius to a player which is then used within a grid system to determine which NetworkObjects are in range. When objects are no longer in the Area of Interest, their network state will no longer be sent and updated on those clients. This feature can be used in all topologies; however, it does have some limitations in Shared Mode, which will be discussed.
Multi-Peer Mode, as opposed to Single Mode, runs multiple clients in a game session without having to run multiple instances of the same build or the Unity editor. Multi-Peer Mode can be setup in a project's Network Project Config Asset
.
Because Area of Interest is best used when there are a lot of NetworkObjects
and players, being able to use it with Multi-Peer mode allows for faster and easier testing of this feature, which this sample demonstrates.
In this sample, the user can generate a specified number of clients. Each client is represented by a spaceship; there are also meteors. Note, there is no collision between ships and/or meteors as the goal of this sample is to focus on implementing Multi-Peer and Area of Interest.
Download
Version | Release Date | Download | |
---|---|---|---|
2.0.0 | Jun 05, 2024 | Fusion Area of Interest Multi-Peer Technical Sample 2.0.0 Build 568 |
Main menu
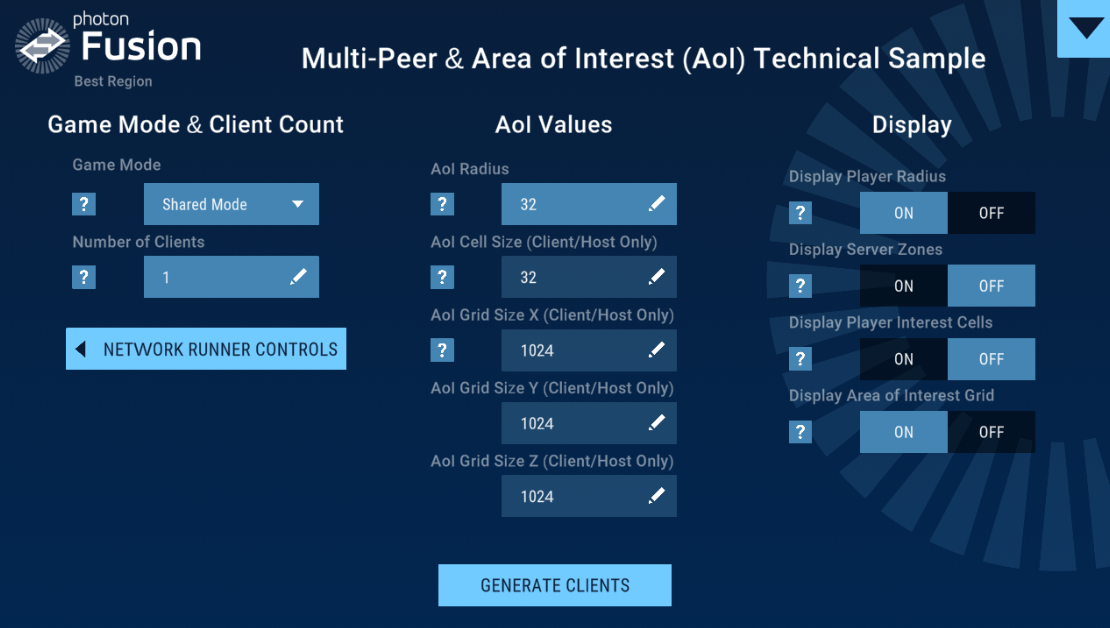
The main menu of this sample contains a lot of different options for users to adjust and test. Note, you can return to this menu at any time by clicking the down arrow in the upper-right of the screen.
- Game Mode & Client Count
- Game Mode: Allows the user to switch between Shared Mode and Client/Host Mode.
- Number of Clients: You can selected how many clients you want to generate when running the sample. Note, you should not exceed your project's number of CCU.
- AoI Values
- AoI Radius: The radius of the player's area of interest. By default this value is 32. In Shared Mode, this cannot exceed 300 units.
- AoI Cell Size: The size of cells in which player AoI is detected, represented by the Server Zones mentioned previously. By default, this value is 32 and cannot be adjusted in Shared Mode.
- AoI Grid Size: The X,Y, and Z size of the overall AoI Grid. By default, this is a 1024 cube and cannot be adjusted in Shared Mode.
- Display
- Display Player Radius: This toggles the player radius used by AoI, represented by yellow, wireframe spheres.
- Display Server Zones: This toggles the Active Server Zones, represented by blue wireframe cubes.
- Display Player Cells: This toggles which cells are currently occupied by players, represented by a cyan highlight within server zones.
- Display Area of Interest Grid: This toggles the entire AoI Grid, represented by a red, wireframe cube.
- Generate Clients: When clicked, this will run a thread that will create and add the specified number of clients to the game.
Network Runner Controls
On the main menu, if the Network Runner Controls
button is clicked, the menu will transition to another containing information similar to that of the Network Runner Controls
window accessible in Unity.
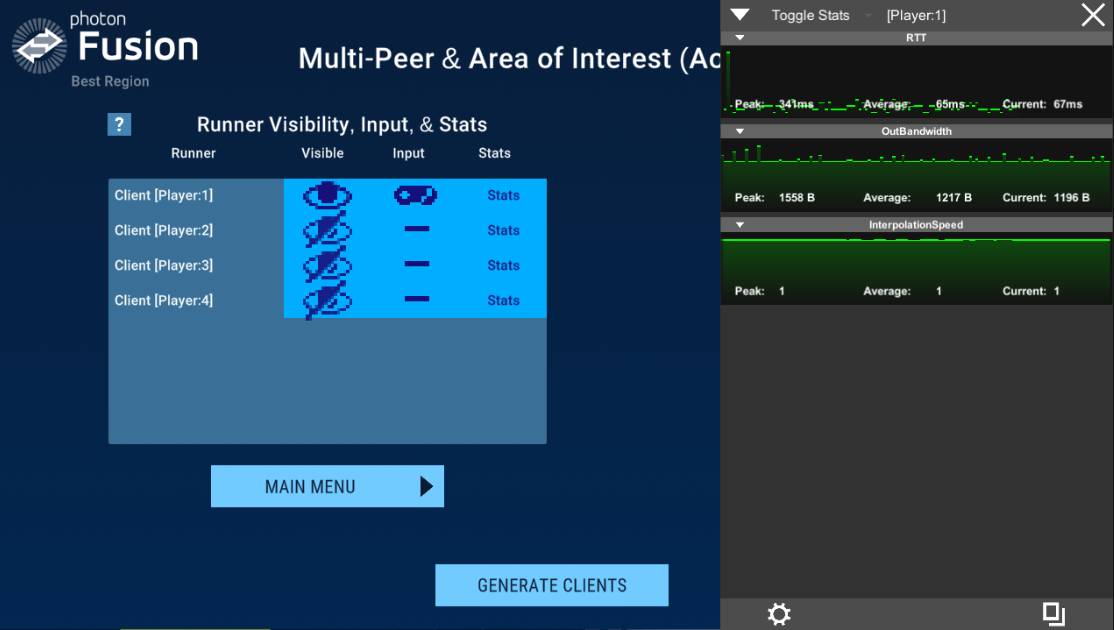
- Runner Visibility, Input, & Stats: Once clients are generated, this list will populate to reflect the number of NetworkRunners available.
- Visibility: Represented by an eye, toggling visibility executed the
NetworkRunner.SetVisiblity
method and will enable or disable various Components set in theEnableOnSingleRunner
Component attached to NetworkObjectss. Note, if this button is left-clicked while holding shift, it will isolate the selected NetworkRunner, hiding the others. - Input: Represented by a gamepad, toggling input sets the
NetworkRunner.ProvideInput
property. When not providing input, theGetInput
method will not detect. This can be done input is only provided for one client. Note, if this button is left-clicked while holding shift, it will turn off input for all NetworkRunner except for the one selected. - Stats: If clicked, this will bring up the Fusion Stats window related to the selected NetworkRunner. You can read more about Fusion Stats here.
- Visibility: Represented by an eye, toggling visibility executed the
Multi-Peer
Network Project Config Settings
It is important to note some changes to the Network Project Config Asset
's default settings. First, for Multi-Peer mode to work, Peer Mode
must be set to Multiple
. Then, Replication Features
must be set to Scheduling and Interest Management
as pictured by the following:
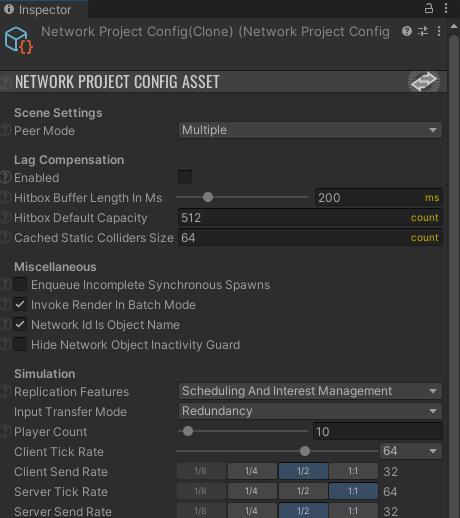
NetworkRunner Setup
For the NetworkRunner
to properly work with Multi-Peer and AoI features, there are a few Components that must be added to the NetworkRunner
prefab that will be instantiated per client.
- Runner Enable Visibility: This will automatically register the associated NetworkRunner with the appropriate extensions and attach loaded scene objects and spawned objects with the peers' visibility handling.
- Runner AoI Gizmos: This Component draw gizmos representing Area of Interest information such as Server Interest Zones and Player Cells. Because it uses gizmos, this information is only visible in the editor.
- Runner AoI Display: This Component was made for this sample specifically and illustrates the same information given through the Runner AoI Gizmos Component, but does so by using a pool of GameObjects to display the previously mentioned information; both this and Runner AoI Gizmos, however, are meant for debugging purposes and should only be used when testing AoI features.
- Fusion Stats: This Component creates a GameObject that displays specific information about this NetworkRunner. It is disabled on start so that it does not open immediately. You can read more about the Fusion Stats tool here.
NetworkObject Setup
By default, when running Multi-Peer Mode, users would see the NetworkObjects
active on every client, regardless of the related NetworkRunner's visibility. To address this, the EnableOnSingleRunner
Component is added to these objects.
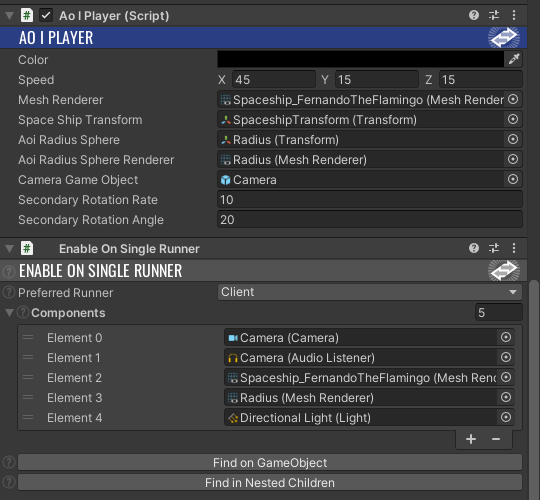
This component is assigned a set of Components that should only be active on visible NetworkRunners. For example, the player ship Light
, Camera
, AudioListener
, and Renderers
will only be visible on NetworkRunners
that are visible. The Preferred Runner set to Client
will allow whichever visible clients to perform this functionality properly.
Generating Clients
Once a user clicks the Generate Clients button on the Main Menu, it'll trigger an async function, BeginMultipeerSession
.
C#
public async void BeginMultipeerSession()
{
mainPanel.SetActive(false);
for (int i = 0; i < PlayersToSpawn; i++)
{
var newRunner = Instantiate(aoiRunnerPrefab);
StartGameArgs startGameArgs = new StartGameArgs()
{
GameMode = currentGameMode,
PlayerCount = 100,
};
var result = await newRunner.StartGame(startGameArgs);
if (!result.Ok)
{
Debug.LogError(result.ErrorMessage);
return;
}
if (newRunner.IsSceneAuthority)
{
var loadSceneResult = newRunner.LoadScene(aoiSceneName, loadSceneMode: UnityEngine.SceneManagement.LoadSceneMode.Additive);
await loadSceneResult;
if (loadSceneResult.IsDone)
{
Debug.Log("Scene Load Complete");
}
}
runnerPanel.AddRunner(newRunner);
}
}
This async function iterates through the number of players specified, starting a game using the GameMode specified on the Main Menu. Once a NetworkRunner has started, if NetworkRunner.IsSceneAuthority
is true, the main scene of the sample will be loaded. Additionally, the visibility and input will be enabled for the first NetworkRunner created, which is done when runnerPanel.AddRunner
is called.
AoI
Settings the AoI Properties
The Area of Interest should only be set within FixedUpdateNetwork
by the NetworkRunner that has State Authority. In this sample, this is done through the following:
C#
...
if ((Runner.IsServer || Object.HasStateAuthority) && !Object.InputAuthority.IsNone)
{
// The player interest must be cleared when no in share mode.
if (Runner.GameMode != GameMode.Shared)
Runner.ClearPlayerAreaOfInterest(Object.InputAuthority);
Runner.AddPlayerAreaOfInterest(Object.InputAuthority, transform.position, MainMenuUI.AreaOfInterestRadius);
}
First, we check if the NetworkObject's NetworkRunner is the server or if the Object has StateAuthority. If true and the InputAuthority has been assigned, we continue. Now, in Shared Mode, the Area of Interest is automatically cleared when assigning; however, in other modes, it must be cleared first. Once this is done, it can be assigned using the current player's InputAuthority, position, and the AoI Radius defined in the main menu.
Setting the Area of Interest Cell and Grid cannot be done in Shared Mode and can only be done by the Server; however, it does not need to be updated every frame, so instead, in the Update
method of the AoIPlayer
class, the following is used:
C#
...
// Only the server can set these items.
if (Runner.IsServer && Object.InputAuthority == Runner.LocalPlayer)
{
if (previousAoICell != MainMenuUI.AreaOfInterestCellSize)
{
Runner.SetAreaOfInterestCellSize(MainMenuUI.AreaOfInterestCellSize);
previousAoICell = MainMenuUI.AreaOfInterestCellSize;
}
if (previousAoIGrid != MainMenuUI.AreaOfInterestGrid)
{
Runner.SetAreaOfInterestGrid(MainMenuUI.AreaOfInterestGrid.x, MainMenuUI.AreaOfInterestGrid.y, MainMenuUI.AreaOfInterestGrid.z);
previousAoIGrid = MainMenuUI.AreaOfInterestGrid;
}
}
This detects if the parameters from the main menu have changed at all, and if so, sets the new values. These settings are set for all players.
IInterestEnter & IInterestExit
When NetworkObjects enter and leave Areas of Interest, their NetworkTransforms and other NetworkProperties will stop being updated on the local client. For example, the meteors will stop moving and then pop to the proper location if they re-enter the area of interest. To prevent this, NetworkBehaviours can implement the IInterestEnter and IInterestExit interfaces, which will trigger upon entering and exiting Area of Interest respectively.
The following is the code used by AoIPlayer
:
C#
public void InterestEnter(PlayerRef player)
{
if (Runner.LocalPlayer != player || !Runner.GetVisible())
return;
gameObject.SetActive(true);
}
public void InterestExit(PlayerRef player)
{
if (Runner.LocalPlayer != player || !Runner.GetVisible())
return;
gameObject.SetActive(false);
}
When an object enters the Area of Interest, we check to make sure the object does not belong to the local player and that the runner is visible. If both are true, the GameObject.SetActive(true)
; the same is performed upon exit, except the GameObject.SetActive(false)
is called.
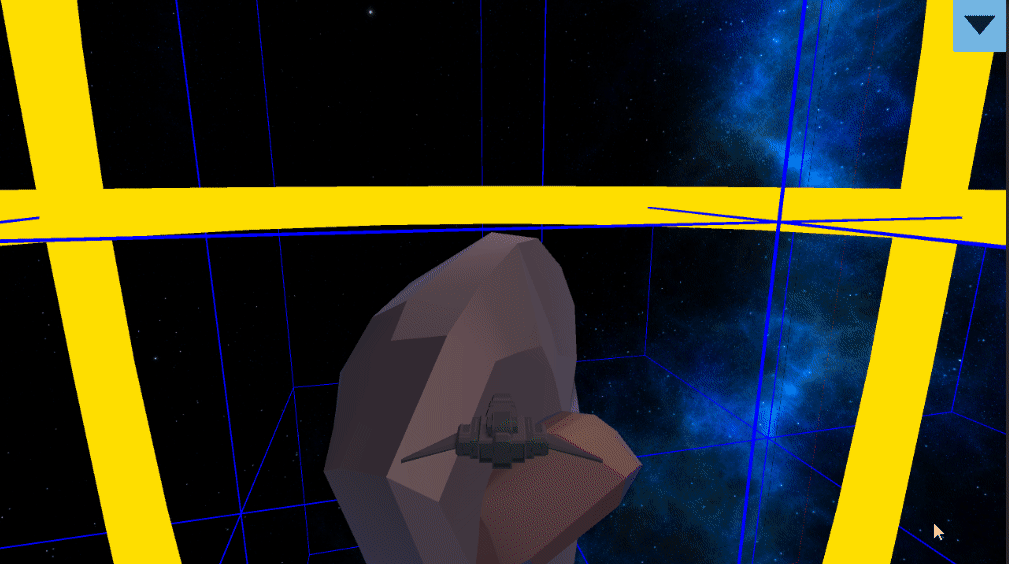
Controls
The following controls will control the ship of any NetworkRunner currently providing input:
- Rotation:
A
andD
- Forward & Back:
W
andS
- Vertical Movement:
Up Arrow
andDown Arrow
Third Party Assets
The spaceship and meteor assets used in this are part of the Ultimate Space Kit created by Quaternius and are licensed through CC0 1.0. The skybox was provided through the following: https://opengameart.org/content/space-skyboxes-0 and is also licensed through CC0 1.0.
Back to top